To connect Django with MongoDB, you can use a Python library called django-mongodb-engine
or djongo
. Both of these libraries provide a MongoDB backend for Django’s Object-Relational Mapping (ORM) layer, allowing you to use MongoDB as your database for Django applications
Install the Library
pip install django-mongodb-engine
Configure Django Settings
In your Django project’s settings.py
file, add the following:
DATABASES = {
'default': {
'ENGINE': 'django_mongodb_engine',
'NAME': 'your_database_name',
'HOST': 'your_mongodb_host',
'PORT': your_mongodb_port,
'USER': 'your_mongodb_username',
'PASSWORD': 'your_mongodb_password',
}
}
Replace the placeholders with your actual MongoDB connection details.
Create Django Models
You can define your Django models as you would normally do with a relational database. The django-mongodb-engine
library will handle the conversion of your models to MongoDB documents.
Perform Database Operations
You can use Django’s standard ORM methods and queries to interact with your MongoDB database, just like you would with a relational database.
Create a new record:
from your_app.models import YourModel
obj = YourModel(field1='value1', field2='value2')
obj.save()
To query the database:
queryset = YourModel.objects.filter(field1='value1')
Saim
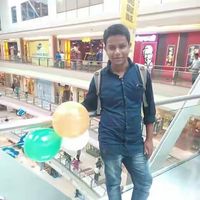
Hi there! My name is Saim and I’m excited to dive headfirst into the world of Python Django. I’m eager to learn and grow every step of the way, and I’ll be documenting my journey through blog posts. I’ll share the highs, lows, and everything in between with you. Together, let’s uncover the wonders of Python Django and its endless possibilities in web development.