In today’s web development landscape, creating visually appealing and functional contact forms is crucial for effective user engagement. This blog post will guide you through the process of building a stylish contact form using Tailwind CSS, a utility-first CSS framework that streamlines the design process.
Getting Started with Tailwind CSS
1. Setup: Begin by including Tailwind CSS in your project. For quick prototyping, use the CDN link:
<script src="https://cdn.tailwindcss.com"></script>
For production, visit Tailwind CSS official website for proper installation instructions.
2. Basic Structure: Start with a simple HTML structure for your form:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Contact Us</title>
<script src="https://cdn.tailwindcss.com"></script>
<script>
function submitForm(event) {
event.preventDefault();
// Add your form submission logic here
alert('Form submitted successfully!');
}
</script>
</head>
<body class="bg-gray-100 min-h-screen flex items-center justify-center p-4">
<div class="bg-white rounded-xl shadow-lg overflow-hidden max-w-md w-full">
<div class="p-8">
<h2 class="text-3xl font-extrabold text-gray-900 mb-6 flex items-center">
<svg class="h-8 w-8 text-indigo-600 mr-2" fill="none" viewBox="0 0 24 24" stroke="currentColor" stroke-width="2">
<path stroke-linecap="round" stroke-linejoin="round" d="M3 8l7.89 5.26a2 2 0 002.22 0L21 8M5 19h14a2 2 0 002-2V7a2 2 0 00-2-2H5a2 2 0 00-2 2v10a2 2 0 002 2z" />
</svg>
Contact Us
</h2>
<form onsubmit="submitForm(event)">
<div class="mb-6">
<label for="name" class="block text-gray-700 text-sm font-semibold mb-2">Name</label>
<div class="relative">
<div class="absolute inset-y-0 left-0 pl-3 flex items-center pointer-events-none">
<svg class="h-5 w-5 text-gray-400" fill="none" viewBox="0 0 24 24" stroke="currentColor">
<path stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="M16 7a4 4 0 11-8 0 4 4 0 018 0zM12 14a7 7 0 00-7 7h14a7 7 0 00-7-7z" />
</svg>
</div>
<input type="text" id="name" name="name" class="pl-10 w-full px-3 py-2 border border-gray-300 rounded-md focus:outline-none focus:ring-2 focus:ring-indigo-500" required>
</div>
</div>
<div class="mb-6">
<label for="email" class="block text-gray-700 text-sm font-semibold mb-2">Email</label>
<div class="relative">
<div class="absolute inset-y-0 left-0 pl-3 flex items-center pointer-events-none">
<svg class="h-5 w-5 text-gray-400" fill="none" viewBox="0 0 24 24" stroke="currentColor">
<path stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="M16 12a4 4 0 10-8 0 4 4 0 008 0zm0 0v1.5a2.5 2.5 0 005 0V12a9 9 0 10-9 9m4.5-1.206a8.959 8.959 0 01-4.5 1.207" />
</svg>
</div>
<input type="email" id="email" name="email" class="pl-10 w-full px-3 py-2 border border-gray-300 rounded-md focus:outline-none focus:ring-2 focus:ring-indigo-500" required>
</div>
</div>
<div class="mb-6">
<label for="message" class="block text-gray-700 text-sm font-semibold mb-2">Message</label>
<textarea id="message" name="message" rows="4" class="w-full px-3 py-2 border border-gray-300 rounded-md focus:outline-none focus:ring-2 focus:ring-indigo-500" required></textarea>
</div>
<div>
<button type="submit" class="w-full bg-indigo-600 text-white font-bold py-3 px-4 rounded-md hover:bg-indigo-700 transition duration-300 flex items-center justify-center">
Send Message
<svg class="h-4 w-4 ml-2 mt-1" fill="none" viewBox="0 0 24 24" stroke="currentColor">
<path stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="M14 5l7 7m0 0l-7 7m7-7H3" />
</svg>
</button>
</div>
</form>
</div>
</div>
</body>
</html>
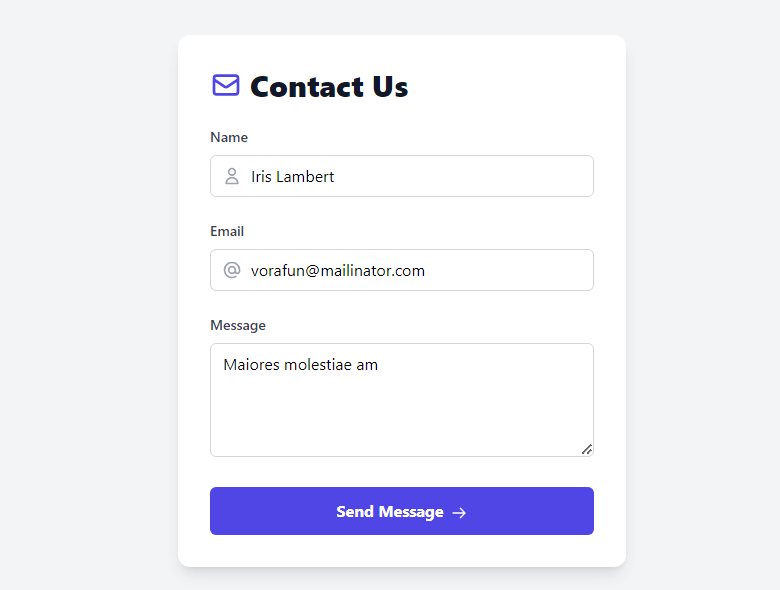
Key Components of the Contact Form
- Input Fields:
- Use
class="w-full px-3 py-2 border border-gray-300 rounded-md"
for consistent styling. - Add
focus:outline-none focus:ring-2 focus:ring-blue-500
for interactive focus states.
- Use
- Labels:
- Style with
class="block text-gray-700 text-sm font-bold mb-2"
for clear, bold labels.
- Style with
- Submit Button:
- Apply
class="w-full bg-blue-500 text-white font-bold py-2 px-4 rounded-md hover:bg-blue-600"
for an attractive, responsive button.
- Apply
- SVG Icons:
- To add a modern touch, let’s incorporate SVG icons from Heroicons. Heroicons offers a set of beautiful, free SVG icons that pair excellently with Tailwind CSS.
Contact Form with Image
<body class="bg-gray-100 min-h-screen flex items-center justify-center p-4">
<div class="bg-white rounded-xl shadow-lg overflow-hidden max-w-4xl w-full flex">
<div class="w-1/2 bg-cover bg-center hidden lg:block" style="background-image: url('https://images.unsplash.com/photo-1486312338219-ce68d2c6f44d?ixlib=rb-1.2.1&auto=format&fit=crop&w=1352&q=80');"></div>
<div class="w-full lg:w-1/2 p-8">
<h2 class="text-3xl font-extrabold text-gray-900 mb-6 flex items-center">
<svg class="h-8 w-8 text-indigo-600 mr-2" fill="none" viewBox="0 0 24 24" stroke="currentColor" stroke-width="2">
<path stroke-linecap="round" stroke-linejoin="round" d="M3 8l7.89 5.26a2 2 0 002.22 0L21 8M5 19h14a2 2 0 002-2V7a2 2 0 00-2-2H5a2 2 0 00-2 2v10a2 2 0 002 2z" />
</svg>
Contact Us
</h2>
<form onsubmit="submitForm(event)">
<div class="mb-6">
<label for="name" class="block text-gray-700 text-sm font-semibold mb-2">Name</label>
<div class="relative">
<div class="absolute inset-y-0 left-0 pl-3 flex items-center pointer-events-none">
<svg class="h-5 w-5 text-gray-400" fill="none" viewBox="0 0 24 24" stroke="currentColor">
<path stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="M16 7a4 4 0 11-8 0 4 4 0 018 0zM12 14a7 7 0 00-7 7h14a7 7 0 00-7-7z" />
</svg>
</div>
<input type="text" id="name" name="name" class="pl-10 w-full px-3 py-2 border border-gray-300 rounded-md focus:outline-none focus:ring-2 focus:ring-indigo-500" required>
</div>
</div>
<div class="mb-6">
<label for="email" class="block text-gray-700 text-sm font-semibold mb-2">Email</label>
<div class="relative">
<div class="absolute inset-y-0 left-0 pl-3 flex items-center pointer-events-none">
<svg class="h-5 w-5 text-gray-400" fill="none" viewBox="0 0 24 24" stroke="currentColor">
<path stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="M16 12a4 4 0 10-8 0 4 4 0 008 0zm0 0v1.5a2.5 2.5 0 005 0V12a9 9 0 10-9 9m4.5-1.206a8.959 8.959 0 01-4.5 1.207" />
</svg>
</div>
<input type="email" id="email" name="email" class="pl-10 w-full px-3 py-2 border border-gray-300 rounded-md focus:outline-none focus:ring-2 focus:ring-indigo-500" required>
</div>
</div>
<div class="mb-6">
<label for="message" class="block text-gray-700 text-sm font-semibold mb-2">Message</label>
<textarea id="message" name="message" rows="4" class="w-full px-3 py-2 border border-gray-300 rounded-md focus:outline-none focus:ring-2 focus:ring-indigo-500" required></textarea>
</div>
<div>
<button type="submit" class="w-full bg-indigo-600 text-white font-bold py-3 px-4 rounded-md hover:bg-indigo-700 transition duration-300 flex items-center justify-center">
Send Message
<svg class="h-4 w-4 ml-2 mt-1" fill="none" viewBox="0 0 24 24" stroke="currentColor">
<path stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="M14 5l7 7m0 0l-7 7m7-7H3" />
</svg>
</button>
</div>
</form>
</div>
</div>
</body>
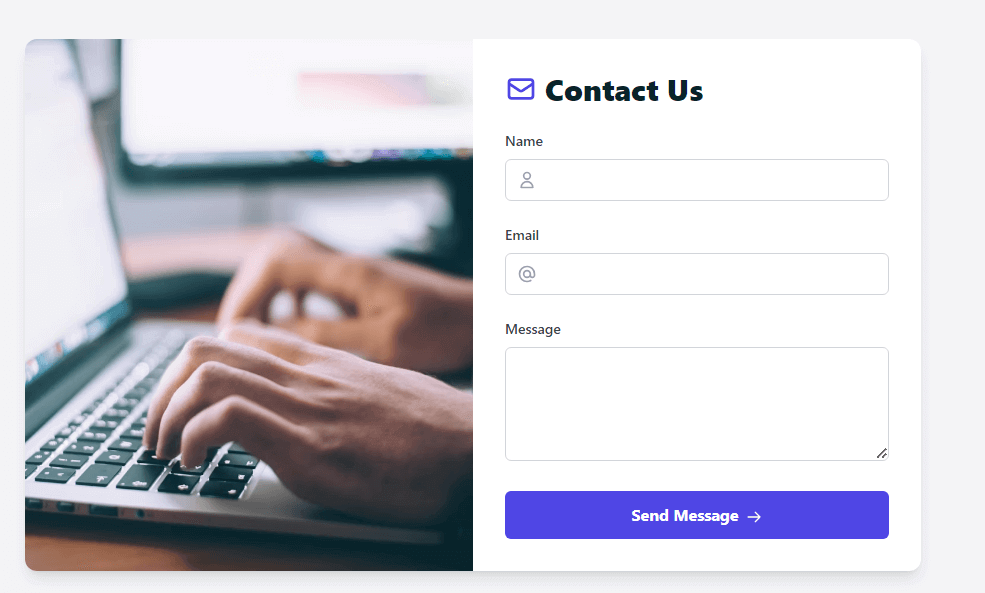
Best Practices
1. Modular Design: Break down your form into reusable components for easier maintenance and consistency across your site.
2. Performance: When moving to production, use Tailwind’s built-in purge feature to remove unused styles and optimize your CSS.
3. Customization: Extend Tailwind’s default configuration to include your custom design tokens for a unique look and feel.