When working with Django, you might start with an abstract base model to share common fields and methods across multiple models. However, there are situations where you might need to convert this abstract model into a concrete one. Whether you’re refactoring your code or adapting your database schema, here’s a step-by-step guide on how to remove the abstract status from a Django model.
Understanding Abstract Models
Abstract models in Django are useful for defining common functionality that you want to share across multiple models without creating a separate database table for the abstract model itself. An abstract model is defined with the abstract = True
option in its Meta
class.
class AbstractModel(models.Model):
name = models.CharField(max_length=100)
class Meta:
abstract = True
AbstractModel
will not have its own table in the database. Instead, it will be used as a base class for other models.
Why Remove the Abstract Status?
There are several reasons you might want to remove the abstract status from a model:
- Refactoring: You might need to make the model concrete to adapt to new requirements.
- Schema Changes: You might want to create a new database table for the model.
- Code Organization: The model might have evolved to be a distinct entity on its own.
Steps to Remove the Abstract Status
A step-by-step guide to converting an abstract model into a concrete one:
1. Update the Model Definition
First, modify the Meta
class in your abstract model to remove or adjust the abstract = True
option.
Original Abstract Model:
class AbstractModel(models.Model):
name = models.CharField(max_length=100)
class Meta:
abstract = True
Updated Concrete Model:
class AbstractModel(models.Model):
name = models.CharField(max_length=100)
class Meta:
# Optionally specify a table name or other options
db_table = 'my_model'
2. Create a New Migration
Django needs to know about the changes to your model, so you need to create a new migration. Run the following command to generate the migration file:
python manage.py makemigrations
This will create a migration file that reflects the changes you’ve made to the model.
3. Apply the Migration
Apply the migration to update your database schema:
python manage.py migrate
This command will apply the migration and create a new table for your model if it wasn’t there before.
4. Update or Create Subclasses
If you have other models inheriting from the abstract model, they might need adjustments. Ensure that these subclasses now conform to the changes you’ve made.
class ConcreteModel(AbstractModel):
additional_field = models.CharField(max_length=100)
Update any references or logic that depends on the abstract nature of the base model.
Conclusion
Removing the abstract status from a Django model can be a straightforward process if you follow the right steps. By updating the model definition, creating and applying a new migration, and adjusting any subclasses, you can successfully transition your model from abstract to concrete.
This change allows you to adapt your models to new requirements and database structures, helping you maintain a clean and efficient codebase.
Saim
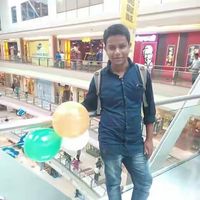
Hi there! My name is Saim and I’m excited to dive headfirst into the world of Python Django. I’m eager to learn and grow every step of the way, and I’ll be documenting my journey through blog posts. I’ll share the highs, lows, and everything in between with you. Together, let’s uncover the wonders of Python Django and its endless possibilities in web development.