When working with Django, the principle of Don’t Repeat Yourself (DRY) can save you time and effort by minimizing redundancy in your code. One of the most effective ways to implement DRY in your Django models is by using abstract base classes. This technique allows you to define common fields and methods once and reuse them across multiple models, promoting cleaner and more maintainable code. In this blog post, we’ll explore how to create and use abstract model classes in Django.
What is an Abstract Model Class?
In Django, an abstract model class is a base class that other models can inherit from. It is defined by setting abstract = True
in the model’s Meta
class. Abstract models are not used to create database tables directly. Instead, they serve as templates for other models that extend them, inheriting fields and behavior.
Benefits of Using Abstract Models
- Reusability: Centralize common fields and methods, reducing code duplication.
- Maintainability: Make changes in one place and have them reflected across all models that inherit from the abstract class.
- Consistency: Ensure consistent behavior and structure across your models.
How to Create an Abstract Model Class
Step 1: Define Your Abstract Model
First, let’s define an abstract model class with some common fields. These fields might include timestamps, flags, or any other attributes shared across your models.
from django.db import models
class BaseModel(models.Model):
created_at = models.DateTimeField(auto_now_add=True)
updated_at = models.DateTimeField(auto_now=True)
is_active = models.BooleanField(default=True)
class Meta:
abstract = True
BaseModel
includes three fields: created_at
, updated_at
, and is_active
. By setting abstract = True
, Django will not create a database table for BaseModel
.
Step 2: Inherit from the Abstract Model
Now that you’ve defined your abstract model, you can inherit from it in your concrete models. This allows your models to automatically include the fields and methods defined in the abstract model.
from django.db import models
from .base import BaseModel # Import the abstract model
class Product(BaseModel):
name = models.CharField(max_length=255)
description = models.TextField()
def __str__(self):
return self.name
class Category(BaseModel):
title = models.CharField(max_length=255)
slug = models.SlugField(unique=True)
def __str__(self):
return self.title
Both the Product
and Category
models inherit from BaseModel
, gaining the created_at
, updated_at
, and is_active
fields without having to redefine them.
Tips for Using Abstract Models
- Multiple Inheritance: If you need a model to inherit from multiple abstract models, ensure there are no field name conflicts and be mindful of Python’s method resolution order (MRO).
- Mixins: Use mixins if you need to share specific behavior or fields across a subset of models, providing more granular control over inheritance.
- Modular Design: Consider organizing abstract models in separate files or modules to promote better project structure and clarity.
Conclusion
Using abstract model classes in Django is a powerful technique for reducing code duplication, promoting consistency, and enhancing maintainability across your project. By defining common fields and methods in abstract models, you can streamline your models and focus on building robust features without getting bogged down by repetitive code. Try incorporating abstract models into your next Django project and experience the benefits of a DRY codebase firsthand.
Saim
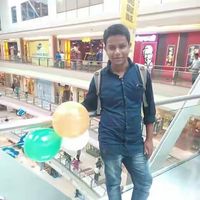
Hi there! My name is Saim and I’m excited to dive headfirst into the world of Python Django. I’m eager to learn and grow every step of the way, and I’ll be documenting my journey through blog posts. I’ll share the highs, lows, and everything in between with you. Together, let’s uncover the wonders of Python Django and its endless possibilities in web development.