In this Django tutorial we will see how to install & setup tailwind flowbit in django app. To use flowbit you need to install tailwind css and django-compressor .
How to Install Tailwind CSS 3 in Django 4
How to Use Tailwind CSS in Django 5
Step 1. Create Django 4 Project
Run below command to create Django project.
django-admin startproject project_name
Step 2. Add Templates Folder in Root Directory
Next, you need to create templates folder in root directory.
|-- project_name
|-- settings.py
|-- templates # new folder
|-- manage.py
Now you need to add templates path in your project_name/setting.py .
# settings.py
TEMPLATES = [
{
...
"DIRS": [BASE_DIR, "templates"], #add templates path
...
},
]
Step 3. Create App For URL and Setup Static Folder
Now you need to create app and static folder for tailwind css assets.
python manage.py startapp pages
Also create static folder and add path in project_name/setting.py Also don’t forget to add pages app in INSTALLED_APPS. Also install django-compressor.
python -m pip install django-compressor
settings.py
# settings.py
INSTALLED_APPS = [
...
'pages', #new
'compressor', # new
]
TEMPLATES = [
{
...
"DIRS": [BASE_DIR, "templates"],
...
},
]
...
COMPRESS_ROOT = BASE_DIR / 'static'
COMPRESS_ENABLED = True
STATICFILES_FINDERS = ('compressor.finders.CompressorFinder',)
Also create src folder and inside create input.css file src/input.css
static
└── src
└── input.css
Now create pages html pages and config URLs.
pages/views.py
from django.shortcuts import render
from django.http import HttpResponse
def index(request):
return render(request, 'index.html')
pages/urls.py
from django.urls import path
from . import views
urlpatterns = [
path('',views.index, name='index')
]
project_name/urls.py
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('', include('pages.urls')),
path('admin/', admin.site.urls),
]
Create all this pages in templates index.html and _base.html.
Step 4. Install Tailwind CSS 3 in Django 4
Run below command to install tailwind css.
npm install -D tailwindcss
Create tailwind.config.js by running below command.
npx tailwindcss init
Now HTML source files to tailwind.config.js.
/** @type {import('tailwindcss').Config} */
module.exports = {
content: ["./templates/**/*.html"],
theme: {
extend: {},
},
plugins: [],
};
Next, you need to Add the Tailwind directives in static/src/input.css.
static/src/input.css
@tailwind base;
@tailwind components;
@tailwind utilities;
Now add npm command in package.json
package.json
{
"scripts": {
"dev": "npx tailwindcss -i ./static/src/input.css -o ./static/src/styles.css --watch",
"build": "npx tailwindcss -i ./static/src/input.css -o ./static/src/styles.css -m"
},
"devDependencies": {
"tailwindcss": "^3.3.3"
}
}
You can run npm command.
npm run dev
# or
npm run build
Step 5. Install Flowbit in Django 4
Install flowbit via npm in django.
npm install flowbite
Add flowbit plugin and path in tailwind.config.js
tailwind.config.js
/** @type {import('tailwindcss').Config} */
module.exports = {
content: ['./templates/**/*.html', './node_modules/flowbite/**/*.js'],
theme: {
extend: {},
},
plugins: [require('flowbite/plugin')],
}
Add tailwind css code and output.css in _base.html.
templates/_base.html
{% load compress %}
{% load static %}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Django + Tailwind CSS + Flowbite</title>
{% compress css %}
<link rel="stylesheet" href="{% static 'src/output.css' %}">
{% endcompress %}
</head>
<body class="bg-green-50">
<!-- Add this -->
<nav class="bg-green-50 border-gray-200 px-2 sm:px-4 py-2.5 rounded dark:bg-gray-800">
<div class="container flex flex-wrap items-center justify-between mx-auto">
<a href="{{ .Site.Params.homepage }}/" class="flex items-center">
<img src="/docs/images/logo.svg" class="h-6 mr-3 sm:h-9" alt="Flowbite Logo" />
<span class="self-center text-xl font-semibold whitespace-nowrap dark:text-white">Flowbite Django</span>
</a>
<button data-collapse-toggle="mobile-menu" type="button" class="inline-flex items-center p-2 ml-3 text-sm text-gray-500 rounded-lg md:hidden hover:bg-gray-100 focus:outline-none focus:ring-2 focus:ring-gray-200 dark:text-gray-400 dark:hover:bg-gray-700 dark:focus:ring-gray-600" aria-controls="mobile-menu" aria-expanded="false">
<span class="sr-only">Open main menu</span>
<svg class="w-6 h-6" fill="currentColor" viewBox="0 0 20 20" xmlns="http://www.w3.org/2000/svg"><path fill-rule="evenodd" d="M3 5a1 1 0 011-1h12a1 1 0 110 2H4a1 1 0 01-1-1zM3 10a1 1 0 011-1h12a1 1 0 110 2H4a1 1 0 01-1-1zM3 15a1 1 0 011-1h12a1 1 0 110 2H4a1 1 0 01-1-1z" clip-rule="evenodd"></path></svg>
<svg class="hidden w-6 h-6" fill="currentColor" viewBox="0 0 20 20" xmlns="http://www.w3.org/2000/svg"><path fill-rule="evenodd" d="M4.293 4.293a1 1 0 011.414 0L10 8.586l4.293-4.293a1 1 0 111.414 1.414L11.414 10l4.293 4.293a1 1 0 01-1.414 1.414L10 11.414l-4.293 4.293a1 1 0 01-1.414-1.414L8.586 10 4.293 5.707a1 1 0 010-1.414z" clip-rule="evenodd"></path></svg>
</button>
<div class="hidden w-full md:block md:w-auto" id="mobile-menu">
<ul class="flex flex-col mt-4 md:flex-row md:space-x-8 md:mt-0 md:text-sm md:font-medium">
<li>
<a href="#" class="block py-2 pl-3 pr-4 text-white bg-green-700 rounded md:bg-transparent md:text-green-700 md:p-0 dark:text-white" aria-current="page">Home</a>
</li>
<li>
<a href="#" class="block py-2 pl-3 pr-4 text-gray-700 border-b border-gray-100 hover:bg-gray-50 md:hover:bg-transparent md:border-0 md:hover:text-green-700 md:p-0 dark:text-gray-400 md:dark:hover:text-white dark:hover:bg-gray-700 dark:hover:text-white md:dark:hover:bg-transparent dark:border-gray-700">About</a>
</li>
<li>
<a href="#" class="block py-2 pl-3 pr-4 text-gray-700 border-b border-gray-100 hover:bg-gray-50 md:hover:bg-transparent md:border-0 md:hover:text-green-700 md:p-0 dark:text-gray-400 md:dark:hover:text-white dark:hover:bg-gray-700 dark:hover:text-white md:dark:hover:bg-transparent dark:border-gray-700">Services</a>
</li>
<li>
<a href="#" class="block py-2 pl-3 pr-4 text-gray-700 border-b border-gray-100 hover:bg-gray-50 md:hover:bg-transparent md:border-0 md:hover:text-green-700 md:p-0 dark:text-gray-400 md:dark:hover:text-white dark:hover:bg-gray-700 dark:hover:text-white md:dark:hover:bg-transparent dark:border-gray-700">Pricing</a>
</li>
<li>
<a href="#" class="block py-2 pl-3 pr-4 text-gray-700 hover:bg-gray-50 md:hover:bg-transparent md:border-0 md:hover:text-green-700 md:p-0 dark:text-gray-400 md:dark:hover:text-white dark:hover:bg-gray-700 dark:hover:text-white md:dark:hover:bg-transparent">Contact</a>
</li>
</ul>
</div>
</div>
</nav>
<!-- End of new HTML -->
<div class="container mx-auto mt-4">
{% block content %}
{% endblock content %}
</div>
<script src="https://cdnjs.cloudflare.com/ajax/libs/flowbite/1.8.0/flowbite.min.js"></script>
</body>
</html>
templates/index.html
{% extends "_base.html" %}
{% block content %}
<h1 class="mb-6 text-3xl text-green-800">Django + Tailwind CSS + Flowbite</h1>
<div class="max-w-sm bg-white border border-gray-200 rounded-lg shadow-md dark:bg-gray-800 dark:border-gray-700">
<a href="#">
<img class="rounded-t-lg" src="/docs/images/blog/image-1.jpg" alt="" />
</a>
<div class="p-5">
<a href="#">
<h5 class="mb-2 text-2xl font-bold tracking-tight text-gray-900 dark:text-white">Noteworthy technology
acquisitions 2021</h5>
</a>
<p class="mb-3 font-normal text-gray-700 dark:text-gray-400">Here are the biggest enterprise technology
acquisitions of 2021 so far, in reverse chronological order.</p>
<a href="#"
class="inline-flex items-center px-3 py-2 text-sm font-medium text-center text-white bg-green-700 rounded-lg hover:bg-green-800 focus:ring-4 focus:outline-none focus:ring-green-300 dark:bg-green-600 dark:hover:bg-green-700 dark:focus:ring-green-800">
Read more
<svg class="w-4 h-4 ml-2 -mr-1" fill="currentColor" viewBox="0 0 20 20"
xmlns="http://www.w3.org/2000/svg">
<path fill-rule="evenodd"
d="M10.293 3.293a1 1 0 011.414 0l6 6a1 1 0 010 1.414l-6 6a1 1 0 01-1.414-1.414L14.586 11H3a1 1 0 110-2h11.586l-4.293-4.293a1 1 0 010-1.414z"
clip-rule="evenodd"></path>
</svg>
</a>
</div>
</div>
{% endblock content %}
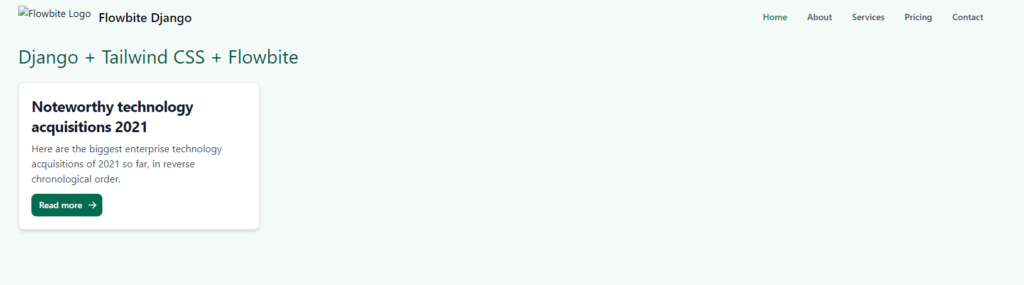
Step 6. Run the Django App Server
Run below command to serve the Django server.
python manage.py runserver