Welcome to our Django tutorial! Today, we’ll show you how to easily add Tailwind CSS 3 to your Django 5 application. Follow along as we use the django-tailwind library to make your web development smoother. If you’re using Django 4, check out the following blog.
How to Install Tailwind CSS 3 in Django 4
Install & Setup Django 5 Project
Run below command to create Django project.
django-admin startproject project_name
Next, create a Django app by running the command below.
python manage.py startapp myapp
Now, include myapp in your settings.py file.
INSTALLED_APPS = [
# other Django apps
'myapp'
]
Next, create HTML pages for your myapp and set up the URL configurations.
myapp/views.py
from django.shortcuts import render
from django.http import HttpResponse
def index(request):
return render(request, 'myapp/index.html')
Make a urls.py file inside the myapp folder.
myapp/urls.py
from django.urls import path
from . import views
urlpatterns = [
path('',views.index, name='index'),
]
Don’t forget to make a templates folder, then inside that, create a folder called myapp and an index.html file.
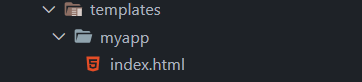
Now import myapp in main project urls.py
project_name/urls.py
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('', include('myapp.urls')),
path('admin/', admin.site.urls),
]
Step 2. Install django-tailwind in Django 5
Install the django-tailwind package via pip.
python -m pip install django-tailwind
If you’d like automatic page reloads while you’re working (check out steps 10-12), use the [reload] option. It’ll install the django-browser-reload package for you.
python -m pip install 'django-tailwind[reload]'
Alternatively, you can install the latest development version via.
python -m pip install git+https://github.com/timonweb/django-tailwind.git
Add tailwind to INSTALLED_APPS in settings.py.
INSTALLED_APPS = [
# other Django apps
'tailwind',
]
Create a Tailwind CSS compatible Django app, I like to call it theme.
python manage.py tailwind init
Add your newly created theme app to INSTALLED_APPS in settings.py.
INSTALLED_APPS = [
# other Django apps
'tailwind',
'theme'
]
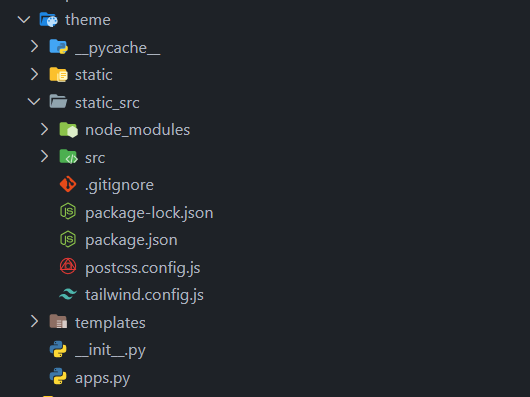
Register the generated theme app by adding the following line to settings.py file.
TAILWIND_APP_NAME = 'theme'
Make sure that the INTERNAL_IPS list is present in the settings.py file and contains the 127.0.0.1 ip address.
INTERNAL_IPS = [
"127.0.0.1",
]
Now Find your pc npm path by running below command.
where npm
Add settings.py file path NPM_BIN_PATH.
NPM_BIN_PATH = 'C:/Users/yourpc/npm.cmd'
Install Tailwind CSS dependencies, by running the following command.
python manage.py tailwind install
The Django Tailwind includes a basic base.html template found at your_tailwind_app_name/templates/base.html. Feel free to customize or remove it if you have your own layout.
If you’re not using the default base.html template from Django Tailwind, just add {% tailwind_css %} to your base.html file.
{% load static tailwind_tags %}
...
<head>
...
{% tailwind_css %}
...
</head>
Next, we’ll include and set up django_browser_reload. It handles automatic refreshing of pages and CSS during development. Just add it to INSTALLED_APPS in your settings.py file.
INSTALLED_APPS = [
# other Django apps
'tailwind',
'theme',
'django_browser_reload'
]
Staying in settings.py, add the middleware.
MIDDLEWARE = [
# ...
"django_browser_reload.middleware.BrowserReloadMiddleware",
# ...
]
Include django_browser_reload URL in your root url.py.
from django.urls import include, path
urlpatterns = [
...,
path("__reload__/", include("django_browser_reload.urls")),
]
myapp/index.html
{% load static tailwind_tags %}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Install Tailwind CSS 3 in Django 5</title>
{% tailwind_css %}
</head>
<body>
<h1 class="text-4xl text-green-500 text-center">Install Tailwind CSS 3 in Django 5</h1>
</body>
</html>
At last, you can now apply Tailwind CSS classes in your HTML. Just start the development server by typing this command in your terminal.
python manage.py tailwind start
For more detailed information, refer to the documentation for the django-tailwind.
[…] Step by Step How to Add Tailwind CSS 3 to Django 5 […]
[…] How to Use Tailwind CSS in Django 5 […]
[…] Step by Step How to Add Tailwind CSS 3 to Django 5 […]
[…] Step by Step How to Add Tailwind CSS 3 to Django 5 […]
[…] Step by Step How to Add Tailwind CSS 3 to Django 5 […]
Superb posts! Have a look at my page Webemail24 where I also put in extra effort to create quality information about Blogging.